Why are React Interviews so Important?
Regardless of the recruitment methods you use for developer search, technical interviews are a must, as they serve as the main approach for identifying the most qualified candidates. And React interviews are not the exception. Below there are three key reasons why you should conduct thorough technical interviews with every relevant candidate.
- To check the candidate's tech skills and experience. According to social psychologist Ron Friedman, 81% of job seekers lie during interviews. Can you imagine how many of them exaggerate their professional competence in CVs? Thus, the first goal of a tech interview (being well-structured and rigorous) is to avoid hiring developers whose experience just doesn’t match your requirements.
- To assess candidates’ analytical, critical, and problem-solving skills. You should be able to fully rely on those React engineers who form the core of your development team. For this reason, make sure you prompt your candidates to think about hypothetical situations that can happen in the workplace to see how they can (potentially) handle them. Their responses will tell you something about crucial soft skills necessary for working in a fast-paced development environment.
- To evaluate candidates in the shortest period of time. Lengthy recruitment processes benefit neither you nor your candidates. The best candidates just won’t hang around why you’re fiddling over a decision. That’s why carrying out a thorough technical interviews is the best option to help you quickly determine a candidate's fit for the position.
In order to make your tech interviews as effective as possible, look for React developers on Huntly! Our two-step screening process with rigorous verification of every CV allows us to present only the most relevant candidates for your open position. As an example, 4 out of 5 Huntly candidates get an interview invitation from employers, which ensures the effectiveness and meaningfulness of every tech interview. Combined with budget-friendly pricing and a personalized approach, our platform is your go-to solution for successful tech recruitment. Don’t waste your precious time — hire with Huntly!
The Most Common React Interview Questions
In order to get the maximum value out of your communication with candidates, check off this list of React technical interview questions for your consideration. Below find widely used React interview questions and answers you could expect to hear from your candidates.
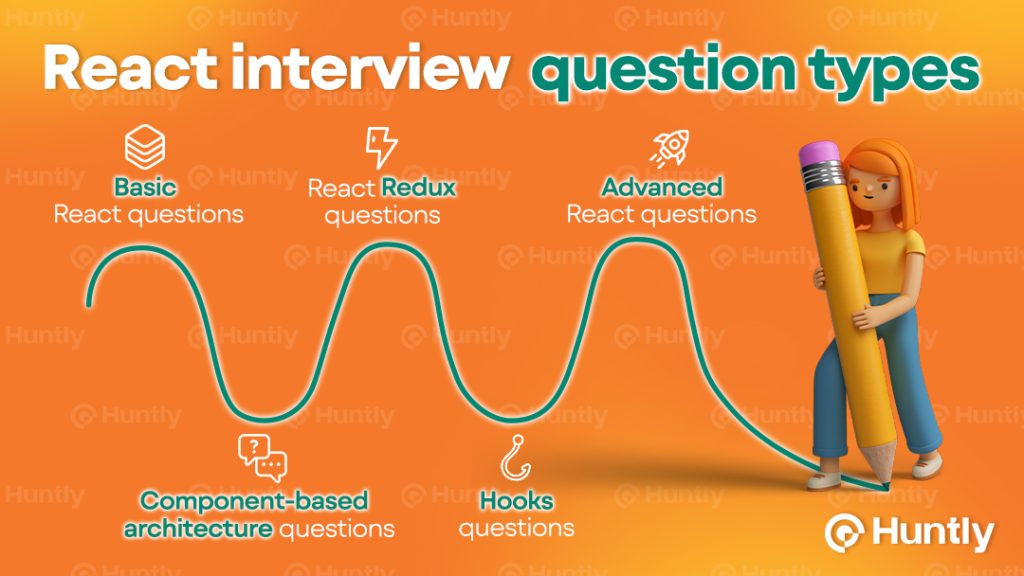
React Basic Interview Questions
In addition to asking what React is, what are its advantages, and how it differs from Angular and Vue.js, you should ask more specific, but still React basics interview questions.
What is Virtual DOM and how does it differ from the real DOM?
Answer to expect: Virtual DOM (Document Object Model) is a lightweight, in-memory representation of the real DOM. Real DOM is a tree-like structure that reflects HTML elements of a web page. The difference between the real DOM and Virtual DOM is the following:
Performance. The Virtual DOM is optimized for performance by minimizing direct manipulations of the real DOM, making updates more efficient.
Batching Updates. The Virtual DOM enables batching of updates, meaning multiple changes can be grouped together and applied at once to minimize the impact on the real DOM.
Reconciliation. The process of comparing the current Virtual DOM with the previous one to determine the minimal set of changes needed for the real DOM is called reconciliation. This is a key feature of the Virtual DOM and helps in optimizing updates.
With that said, the Virtual DOM acts as an intermediary between the application's logic and the actual browser DOM, providing a more efficient way to handle updates and improve the performance of web applications, especially those with dynamic content and frequent UI changes.
What are events in React?
Answer to expect: Event in React is a response to any interaction with a website's UI such as clicking a button, typing in an input field, hovering a mouse, etc. Events in React are handled with event handlers: functions that are executed when a specific event occurs. Common React events include mouse events, keyboard events, form events, etc.
What are the main building blocks in React?
Answer to expect: Building blocks, or components, are reusable pieces of code that can be of two types: functional and class components. Functional components are simple components that don't hold any internal state. They get data from their parent components through props and return JSX to describe what should be shown on the screen. Class components are more complex: they have their own internal state and lifecycle methods, allowing them to manage data and respond to changes during their lifecycle.
What is JSX?
Answer to expect: JSX stands for JavaScript XML, a special add-on to JavaScript that lets developers mix HTML-like syntax right into their JavaScript code. With JSX, it’s possible to create components using a blend of JavaScript and HTML, making code much simpler to read and grasp.
What debug tools for React do you know?
Answer to expect: There are several debug tools that can be used to improve the quality of React code: React DevTools, Redux DevTools Extension, React Developer tools in Chrome DevTools.
Component-Based Architecture Questions
One of the distinctive characteristics of React is its component-based architecture, so you should ask some common React interview questions about components.
What’s the difference between props and state for component communication?
Answer to expect: Props (or properties), allow data to be transferred from a parent component to a child component in React. Once passed to a component, props cannot be altered as they are immutable. State, on the other hand, embodies the internal condition of a component. It's like a container holding data that can be modified within the component itself.
What does the component lifecycle look like in React?
Answer to expect: The component lifecycle consists of three stages: mounting, updating, and unmounting. During the mounting stage, a component is being put into the DOM. At the updating stage, the component is updated if there’s a change in state or props. The last stage aims to remove the component from the DOM.
What are HOCs in React and why use them?
Answer to expect: HOCs (high-order components) are functions aimed at reusing component logic. HOCs substitute components with new components allowing for adding new functionality without changing the components’ code. HOCs can also be used for bootstrap abstraction, state manipulation and abstraction, manipulation of props, and render hijacking.
React Redux Interview Questions
React is often used with Redux, so you should definitely ask some React Redux interview questions when talking to candidates.
What is Redux?
Answer to expect: Redux is a library for state management that helps developers build consistent apps that will behave the same in different environments.
What are the core concepts of Redux?
Answer to expect: The core Redux concepts include actions, reducers, and store. Redux actions are used to send data from an app to the Redux store. Redux reducers manage the way application state changes as a result of particular actions. Redux store is a JavaScript object that holds the application state and dispatches actions to reducers with dispatch(action).
What are the benefits of using Redux with React?
Answer to expect: When using Redux with React, one benefits from an organized app structure where every change is predictable. Moreover, Redux improves app performance, thus adds to the ease of debugging and maintainability.
Hooks Questions
Questions about Hooks are among the top React interview questions that allow you to dig deeper into candidates’ understanding of the technology.
What are React Hooks?
Answer to expect: Hooks are functions that enable you to tap into React's state and lifecycle functionalities directly from functional components. They offer a means to utilize state and other React capabilities without the need to employ class components.
What are the most used Hooks in React?
Answer to expect: The most common React Hooks are useState, useEffect, useContext, and useRef. useState allows functional components to have a state. useEffect is responsible for side effects in functional components. useContext allows for using context in functional components. useRef generates a mutable ref object that remains consistent across renders.
React Advanced Interview Questions
If you’re looking for a senior React developer, you need to challenge them with hard interview questions. Below are some examples of React advanced interview questions to use during tech interviews with candidates for senior and lead positions.
How would you improve the performance of a React app?
Answer to expect: In order to enhance the performance of React applications, one can employ strategies such as utilizing React.memo() to prevent unnecessary re-renders of components, useCallback() and useMemo() to cache functions and values, and implementing lazy loading and code splitting to mitigate initial loading durations.
Why would you use refs in React?
Answer to expect: Refs in React are mainly used for three reasons: 1. to initiate imperative animations; 2. to join third-party DOM libraries; 3. to manage focus and apply media playback.
What’s the purpose of a router in React?
Answer to expect: In React, a router plays a crucial role in managing various routes whenever a user enters a URL. If the router contains a route corresponding to the entered URL, the user is directed to that specific route. To enable this functionality, the router library must be integrated into React.
To get the most out of a tech interview, you can also ask candidates React coding interview questions according to their level. At this stage, you can challenge candidates with tasks like identifying the purpose of a particular code, finding an error in the code, explaining why using this or that function is (in)appropriate in a piece of code, etc. It’s even better to test candidates’ coding skills during an interview. According to Stack Overflow, one hour of practical coding will tell you more about a candidate's hard skills than one hour of asking questions.
Interview Questions for React Developers to Check Soft Skills
Checking technical proficiency is essential when choosing among candidates, but you shouldn’t limit your interviews to tech questions only. Make sure there are some interview questions for React developers that delve into their soft skills such as teamwork, time management, problem-solving skills, critical/analytical thinking skills, and creativity.
Find examples of questions you can use to understand each of these traits in the table below.
Soft skill to check | Question to ask |
Teamwork | - Are you an independent worker or a team player? - If you had a conflict with your team member, how would you manage it? - How would you act if your manager didn’t agree with your decision? |
Time management | - How do you prioritize several tasks with the same deadline? - How do you usually organize your working day? - How do you evaluate the time you need to spend on a task? |
Problem-solving | - What was your biggest coding challenge and how did you solve it? - What would you do if a client wasn’t happy with the results of your work? - Describe a situation when you had to make an important decision without your manager. |
Creativity | - Is creativity important for a React developer? - Describe a situation when you had to think out-of-the-box. - Have you ever integrated a new idea or approach into your routine work process? |
With this list of interview questions for React developers, you’ll be able to get the most out of technical interviews as well as learn the maximum about candidates’ personalities.
You can also save time and costs by holding interviews with Huntly candidates! We specialize in sourcing top tech specialists all over the world. Post your vacancy on our recruitment platform and get a pipeline of pre-vetted React developers within 72 hours.
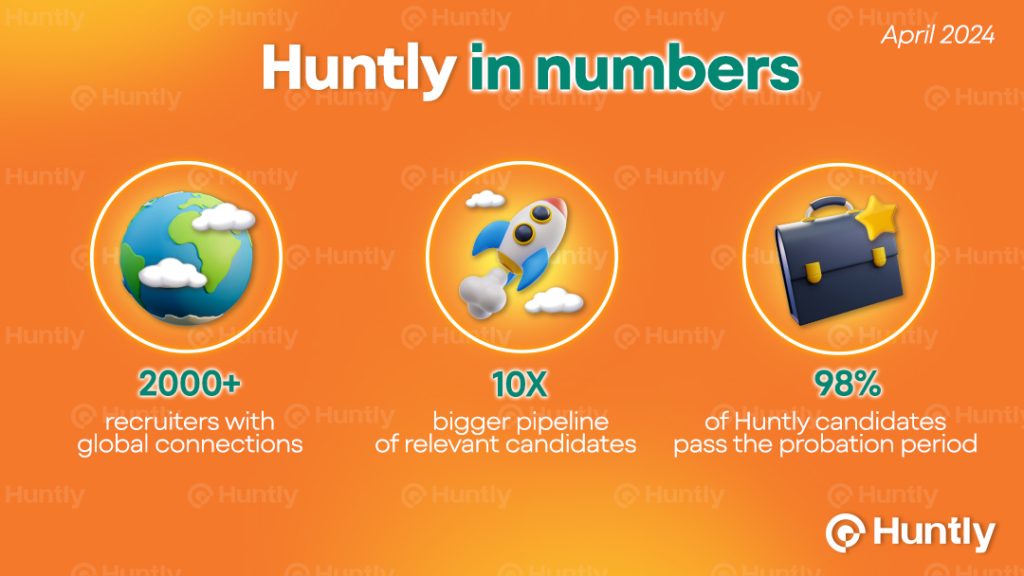